8.12
Problem set 2: Robert Indiana🔗
This assignment is due on Wednesday, January 17 at 11:59pm. Submit it using Handin as assignment ps2.
Your submission is only accepted if the message “Handin successful” appears.
Corrections can be presented until Friday, February 9.
This problem set builds on the skills that we introduced in
Lecture 2.
To encourage you to review that lecture, your grade on this
assignment will be capped by your grade on that lecture
at the time you submit (or correct) this assignment. You can resubmit
lecture exercises at any time.


1 Warmup: calculating numbers🔗
In every problem set, clearly delimit your work for each exercise using a comment like “; Exercise 1”.
Exercise 1.
Define the variable x to be a big number.
Then define the variable y to be the cube of x.
Put digits only in your definition of x, not in your definition of y.
(If you’re not sure how to define a variable, review Lecture 2: Definitions Exercise 1.)
Exercise 2.
In comments, show step-by-step calculations that lead from your definition of y to its computed numeric value.
Show a separate step for each time your definition of x is used, and for each time a bigger number is produced.
So, your calculations should include at least 2 steps (depending on how many times your definition of y uses x).
(If you’re not sure how to calculate step-by-step, review Lecture 1: DrRacket and arithmetic Exercises 10 and 13 and Lecture 2: Definitions Exercise 3.)
Exercise 3.
Define a function cube that takes a number x as input and returns its cube.
We’ve already given the signature and purpose for the function:
; cube : Number -> Number |
; produce the third power of the given number |
Here are some examples of using cube:
; (cube 4) should be 64 |
; (cube 1) should be 1 |
And your definition should look like this:
(define (cube x) |
FILL-IN-THIS-BLANK) |
(If you’re not sure how to define a function, review
Lecture 2: Definitions Exercise 5.)
Use your new function to compute the cube of 10.
Exercise 4.
Let us compute the cube of the cube of the cube of 3.
In comments, show step-by-step calculations that begin with
; (cube (cube (cube 3))) |
; = FILL IN REMAINING STEPS |
Show a separate step for each time your definition of
cube is used, and for each time a bigger number is produced.
So, your calculations should include at least 6 steps.
(If you’re not sure how to calculate step-by-step, review
Lecture 2: Definitions Exercise 8.)
2 Eat/Die🔗
The famous “Love” design above was created by the artist Robert Indiana (born
in New Castle, Indiana in 1928; died in Vinalhaven, Maine in 2018). In the
rest of this problem set, you will use definitions to roughly recreate some
other works by him. Throughout this problem set, do not copy, paste, or edit
any picture at any time in DrRacket; in other words, never put any made picture
in your Definitions Window (except perhaps in comments).
Robert Indiana often paired the words “eat” and “die”, because “eat” was
the last word that his mother said before she died.
Exercise 5.
Define the variable
diamond-eat to be an image similar to the left
half of the diptych above. To do so, use the
overlay function
provided by the
2htdp/image library to combine three images:
a dark green text image, produced using the text function,
a solid red circle, produced using the circle function, and
a dark green diamond, produced either using the rhombus function
or using the rotate and square functions.
“las complejidades de nuestro ser, su fuego y su álgebra”
—Jorge Luis Borges
A computer scientist might diagram the operations as follows:
When you hit the “Run” button, then in the Interactions Window after the
prompt “>” type diamond-eat and hit Enter, DrRacket should
respond with some picture like this:
Hint: First make the three ingredient images in the Interactions Window, then
feed them to the
overlay function in the Definitions Window.
Throughout this course, when we tell you to define something and specify the
name (such as diamond-eat), you must define it with exactly that name.
Other names such as diamondeat or diamond-Eat are not
acceptable.
Note: On some computers, the text may be off center because of font differences.
Don’t worry about it! If it really bugs you, you can try using the
text/font function to choose a different font.
Exercise 6.
Define a function diamond that takes a string and returns an image of
it inside a circle inside a diamond. Your function should have this signature and purpose:
; diamond : String -> Image |
; draw a diamond with the given text inside |
|
; (diamond "EAT") should be the picture above |
For example, when you hit the “Run” button, then in the Interactions Window
after the prompt “>” type (diamond "EAT") and hit Enter,
DrRacket should respond with the same picture as above. Check that,
then change your definition of diamond-eat to
(define diamond-eat (diamond "EAT")) |
without affecting the image value of diamond-eat.
Exercise 7.
Define the variable
diptych to be an image similar to Robert Indiana’s
diptych above. To do so,
use your
diamond function twice, and
combine the resulting images using the
beside function provided by the
2htdp/image library.
A computer scientist might diagram the operations as follows:
The diagram matches these signatures:
; diamond : String -> Image |
; beside : Image Image -> Image |
Remember that
using something means putting its
name in your
code, so your definition of
diptych should contain the name
diamond twice, and not the names
overlay or
text or
circle. Again, your definition should not contain any picture either.
When you hit the “Run” button, then in the Interactions Window after the
prompt “>” type diptych and hit Enter, DrRacket should respond
with some picture like this:
Don’t worry about the color mismatch between Robert Indiana’s work and our
crude approximation. Feel free to change the colors in your diamond
function, but it would affect both diamonds at once.
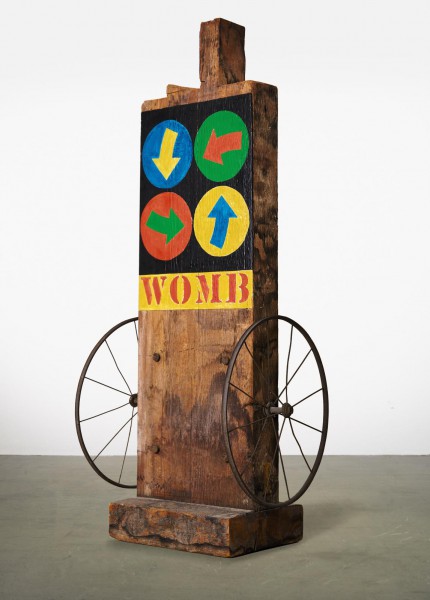
Exercise 8.
Define the variable
blue-arrow-on-yellow to be an image similar to the
lower-right arrow on the sculpture above. To do so, use the
overlay
and
above functions provided by the
2htdp/image
library to combine three images:
A computer scientist might diagram the operations as follows:
When you hit the “Run” button, then in the Interactions Window after the
prompt “>” type blue-arrow-on-yellow and hit Enter, DrRacket
should respond with some picture like this:
If you see a thin seam between the triangle and the rectangle, don’t worry about it.
Hint: Like with arithmetic on numbers, the order and grouping of operations matters.
Exercise 9.
Define a function arrow that takes two colors and returns an image of
an arrow of the first given color inside a circle of the second given color.
Your function should have this signature and purpose:
; arrow : Color Color -> Image |
; draw an arrow of the first color inside a circle of the second color |
|
; (arrow "blue" "yellow") should be  |
For example, when you hit the “Run” button, then in the Interactions Window
after the prompt “>” type (arrow "blue" "yellow") and hit
Enter, DrRacket should respond with the same picture as above. Check that,
then change your definition of blue-arrow-on-yellow to
(define blue-arrow-on-yellow (arrow "blue" "yellow")) |
without affecting the image value of blue-arrow-on-yellow.
Exercise 10.
Use your
arrow function and the
rotate function
provided by the
2htdp/image library to recreate the upper-right
arrow on the sculpture:
The diagram matches these signatures:
; arrow : Color Color -> Image |
; rotate : Number Image -> Image |
Again, if you see a thin seam between the triangle and the rectangle, don’t worry about it.
Exercise 11.
Define the variable womb to be an image similar to the painted arrows
on Robert Indiana’s sculpture above. To do so, use your arrow
function 4 times and the rotate function 3 times, and combine the
resulting images using the beside and above functions
provided by the 2htdp/image library. Again, using
something means putting its name in your code, so your definition of
womb should not contain overlay or triangle or
rectangle or circle or any picture.
When you type womb in the Interactions Window, DrRacket should respond
with some picture like this:
Hint: Diagram the operations; plug together these signatures:
; arrow : Color Color -> Image |
; rotate : Number Image -> Image |
; beside : Image Image -> Image |
; above : Image Image -> Image |
4 Extra fun🔗
Help other students by answering this ungraded question: what did you have to
learn to finish this problem set that we didn’t teach? Post your answer to
Discord in the #ps2 channel, or put it as a comment at the bottom of your
Handin submission.
Students in H211 should complete all the Extra fun exercises;
they are optional and not graded for students in C211.
Exercise 12.
Recreate The Electric American Dream:
Note that the bottom of the “Hug” circle descends below the top of the
“Eat” and “Die” circles, and the top of the “Err” circle rises above the
bottom of the “Eat” and “Die” circles. This placement can be achieved using
rotate or
place-image, but there are other ways as well.
Exercise 13.
Recreate The Triumph of Tira:
Your code must use
star in just one place, and
circle in at most two places.
Unlike with Eat/Die above, do capture how the 4 circles
are colored differently.

Exercise 14.
Many flags are triband, meaning that they are composed of three
parallel bands of color. For example, the flag of France has three
vertical bands, whose colors are dark blue, white, and red. With this
flag as with many other flags, the three bands are equally wide, and
together they form a rectangle that is 150 wide and 100 tall.
Define a function vertical-triband that takes three colors as
inputs and produces a vertical triband flag image.
For example, (vertical-triband "dark blue" "white" "red")
should produce the flag of France.
Hint: before defining a function, first produce a flag.
Exercise 15.
Some triband flags have horizontal rather than vertical bands.
For example, the flag of the Netherlands has three horizontal bands,
whose colors are dark red, white, and dark blue.
Use vertical-triband to define a function horizontal-triband that takes three colors as inputs and produces a horizontal triband flag image.
For example, (horizontal-triband "dark red" "white" "dark blue")
should produce the flag of the Netherlands.
Hint: besides vertical-triband, also use scale/xy and rotate.