8.12
Lecture 12: Unlimited data
This assignment is due on Tuesday, February 13 at 11:59pm. Submit it using Handin as assignment lecture12.
1 Pre-flight check
Check your answer using “Show answer” above. Did you get it right? If not, click the button again to get another set, and keeping doing it until you get it right without peeking at the answer.
You don’t need to submit anything for this check.
But the longer you put off mastering the skill of writing templates,
the harder the rest of this entire course will be for you.
The first video of Lecture 11: More points recaps how to write templates (for processing unions).
More details and exercises are found in
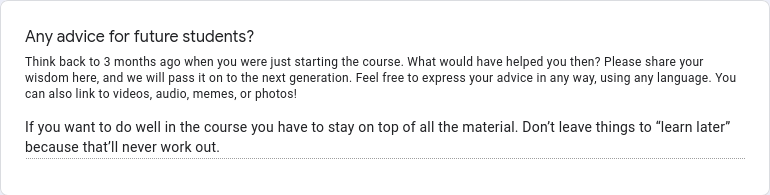
Lecture 10: Unions of structures and Lab 5: Unions for unions,
Lecture 8: Structures and Lab 4: Structures for structures, and
Lecture 6: Multiple cases and Lab 3: Multiple cases for enumerations.
2 Unlimited data
; A Point is (make-point Number Number) (define-struct point [x y]) ; A BunchOfPoints is one of: ; - (make-none) ; - (make-some Point BunchOfPoints) (define-struct none []) (define-struct some [first rest])
Exercise 1. Define 3 examples of BunchOfPoints. Don’t use the examples from the video.
Exercise 2.
Copy the comments below to your Definitions Window. For each of the 6
expressions, say whether it is a BunchOfPoints, and explain why not if
not. The first 2 parts are already done for you; follow their pattern.
; 1. (make-some (make-point 1 1) (make-none)) ; is a BunchOfPoints ; 2. (make-point (make-some 1 1) (make-none)) ; is not a BunchOfPoints because it is a ; use of neither make-some nor make-none ; 3. (make-some (make-point 70 40) ; (make-point 30 50)) ; ; 4. (make-none) ; ; 5. (make-some (make-point 30 50) ; make-none) ; ; 6. (make-some ; (make-point 70 40) ; (make-some (make-point 30 50) ; (make-none))) ;
Exercise 3.
Here’s the first two steps of the design recipe for count-all-points.
; count-all-points : BunchOfPoints -> Number ; count how many points are in the bunch (define (count-all-points bop) ...)
Write the template for count-all-points.
Make it look like a function called process-bop,
and do not put it in a comment.
Exercise 4.
Finish designing count-all-points.
Exercise 5.
Finish designing this function:
; move-points-up : BunchOfPoints -> BunchOfPoints ; subtract 1 from every Y coordinate ; in the given BunchOfPoints (check-expect (move-points-up (make-none)) (make-none)) (check-expect (move-points-up (make-some (make-point 30 50) (make-none))) (make-some (make-point 30 49) (make-none))) (check-expect (move-points-up (make-some (make-point 70 40) (make-some (make-point 30 50) (make-none)))) (make-some (make-point 70 39) (make-some (make-point 30 49) (make-none)))) (define (move-points-up bp) ...)
Exercise 6.
Using the functions you’ve defined, and the mouse
function defined in the video, run the big-bang animation
shown.
Exercise 7.
Optional: Change mouse to only add
points when you click the mouse, as shown at the end of the video.