when the browser menu is displayed.
There are several functions built into the editor
which allow for the debugging of microcode programs.
These are listed in Table III.
Table III. Debugging Functions
Function
| Key Binding
| Description
|
Run
| A-F6
| Put the 2910 into run mode.
It will run at the rate of the LE Board clock.
|
Single Step
| F6
| Execute one instruction of the microcode.
|
Idle
| C-F6
| Take the 2910 out of run mode.
|
Run Breakpoint
| A-F7
| Put the 2910 into run mode and wait for a breakpoint.
|
Toggle Breakpoint
| F7
| Set/Clear a breakpoint for the current instruction.
|
Display 2910
| A-F8
| Toggle the display of the 2910 window.
|
Clear 2910
| C-F7
| Reset the 2910.
|
Once a microassembly program has be successfully assembled and
downloaded, it can be run from the LEASMB environment.
In the
most simple case, the program can be started, stopped, and restarted.
To start the program running, use the
Run
function.
This will
start the program from the current location (highlighted) and
run at the rate of the LE board clock.
When the program is running,
there is no feedback to the software.
To stop the program from
running, use the Idle function.
This will stop the execution
of the 2910 and update the current location to the instruction
where the program was stopped.
The Clear 2910 function will reset
the contents of the 2910 and thus reset the microprogram to be
started at location 0.
The
Single Step
functions causes one microinstruction to be executed.
After execution, the cursor will be relocated to the next instruction
to be executed and this instruction will be highlighted.
The
Toggle Breakpoint
function allows the user to set or clear
a breakpoint on any microinstruction.
In order to set a breakpoint,
the microcode must have been successfully assembled.
If the code
has been assembled, but the LE board is not responding, a bell
will be sounded when the Toggle Breakpoint function is used, indicating
that the breakpoint was not downloaded to the LE Board.
Breakpoints
are used in conjunction with the
Run/Breakpoint
function which
is used to put the LE Board into run with breakpoint mode.
The
Run/Breakpoint
function is similar to the Run function with
the exceptions that none of the other LE ASMB functions are available
while in run with breakpoint mode.
The board will stay
in this mode until an instruction with a breakpoint set is to
be executed or the cancel field is selected in the dialog box
that is displayed upon entering run with breakpoint mode.
When the board leaves this mode, the dialog box will be closed,
the cursor will be moved to the current microinstruction, and
the current microinstruction will be highlighted.
The
Display 2910
function allows the user to observe the contents
of the 2910 while debugging.
This function will toggle the display
of the 2910 window.
When displayed, this window contains the
values of the I and D inputs to the 2910 and the
contents of the PC register, R register and the
Stack of the 2910.
The values in this window are updated
whenever the execution of the 2910 is stopped as after an
Idle,
Single Step
or
Clear function.
The window will not continuously
update while the 2910 is running.
Modern control microprograms usually have complex
responsibilities, and the code is often quite complicated.
We developed the LEASMB Microprogram Assembler with the hardware
designer's needs in mind.
We strove for simplicity and for a
small set of powerful functions that support structured design.
Before describing the details of the LEASMB language, we will
present a design illustration to give the flavor of the language
and its use.
Hardware designers separate a design problem into
an architecture (the registers, data paths, etc) and a control
algorithm (for our purposes, a microprogram).
The control program
receives status information from the architecture, and delivers
command information to the architecture.
The command information
is contained in the microinstruction, along with information governing
the sequence of microinstructions.
The microprogram assembler
must give the designer a flexible and powerful language in which
to express the command and sequencing information.
The 2910
microprogram sequencer is at the core of Logic Engine control,
and in this manual we will assume that you are familiar with this
chip.
Even so, since we present this example to familiarize you
with the style of the LEASMB assembler, not the 2910, you should
have little difficulty even if you are not yet familiar with the
2910.
To eliminate unwanted detail, yet provide a real
design example, our model uses hardware extracted from a larger
design -- a high-speed stack-oriented computer.
Our microprogram illustrates the control of a small set of tasks
required in the debugging of this hardware.
Figure 1 shows the
architecture for our illustration.
We focus on the top three
elements of the main stack.
The larger task involves various
movements of the data among these stack elements, but our illustration
will deal with two operations: (a) a load operation that pushes
new external data onto the stack for debugging purposes, and (b)
a cyclic rotation of the top three stack elements.
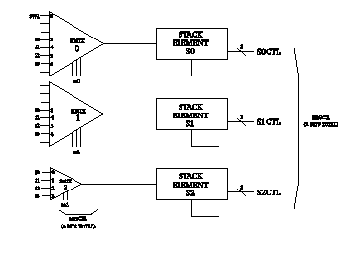
Figure 14. LE ASMB design example: Architecture
Figure 15. LE ASMB design example: Test input selection
Each stack element (which may contain as many bits as necessary)
receives its input from a multiplexer (actually, a set of multiplexers,
one for each bit, controlled identically).
Each desired source for a given stack element becomes an input
to that element's multiplexer.
For testing purposes, in addition to the stack elements, inputs
include the switch register on the display panel.
We shall call
the select signals for the multiplexers M0, M1, and M2, and we
shall refer to the entire collection of multiplexer select signals
as MUXCTL.
In the full design, each stack element requires two
control inputs; we call these pairs of bits S0CTL, S1CTL, and
S2CTL, and we call the collection of stack element controls REGCTL.
To direct the actions of the 2910, the designer will usually need
to make available several status signals from the architecture.
The 2910 sequencer accepts a single signal as a test input.
2910 instructions may interrogate this signal and branch based
on its value.
The designer of a microprogrammed system faces
the problem of extracting the desired signal from the architecture
and presenting it to the sequencer at the proper time.
Of the several mechanisms for selecting one signal from many, perhaps
the easiest and simplest is to construct a multiplexer in the
architecture.
Since in each microinstruction we know which signal,
if any, is required for testing, we may use some of the command
bits in the microinstruction to serve as the select code for the
multiplexer.
The full design from which this example is taken
requires about a dozen test inputs, so a 16-input multiplexer
with a four-bit select code is appropriate.
Figure 2 shows
the structure of the test input selection apparatus, centered
around the test multiplexer INMUX.
You will notice that we have
decided to allocate the first four microinstruction command bits
(bits 0-3) to control the test multiplexer.
This is an arbitrary choice.
Developing the Control Program
An LEASMB microprogram assembly has two parts.
In the declaration phase,
we specify symbolic names for all variables and quantities
of interest, and we describe the structure of the microinstruction.
The program phase begins with the directive
PROG
and contains the microcode itself, in symbolic form.
A microinstruction has
a sequencer part, which drives the 2910 and determines the address
of the next microinstruction, and usually a command part, in which
the programmer specifies values for command signals that control
the architecture.
ID LEASMB_demo
* SAMPLE DECLARATIONS AND SAMPLE MICROCODE
SIZE 23; number of command bits
MODE LOGIC
* COMMAND FIELD DECLARATIONS
MUXCTL(10:0) COM (4:14),T=$7FF,D=%TTTTTTTTTTT
M0(2:0) EQU MUXCTL(9:7); mux 0 select signals
M1(2:0) EQU MUXCTL(6:4); mux 1 select signals
M2(1:0) EQU MUXCTL(3:2); mux 2 select signals
M0S2 INV M0=5; select reg S2 through mux 0
M0SWR INV M0=0; select switch reg through mux 0
M1S0 INV M1=3; select reg S0 through mux 1
M2S1 INV M2=1; select reg S1 through mux 2
REGCTL COM (15:22), T=%HHHHHHHH,D=%FFFFFFFF
LOAD3 EQU %11111100; load S0,S1,S2
ROTATE INV M0S2,M1S0,M2S1,REGCTL=LOAD3; rotate stack
* INPUT TEST MUX CONFIGURATION (2 input signals defined)
INMUX(3:0) COM (0:3),T=%HHHH,D=0
LD.L INV INMUX=0,T=%L
TST.L INV INMUX=1,T=%L
PROG
LOC XDDDI CCCC CC
000 ORG 0
000 BEGIN EQU *
000 10033 0FFE 00 LOAD JUMP TEST IF LD.L=%F
001 50013 0FFE 00 JUMP * IF LD.L=%T
JUMP BEGIN;M0SWR,M1S0,M2S1,
002 30003 086F F8 REGCTL=LOAD3; push switches onto stack
003 10003 1FFE 00 TEST JUMP LOAD IF TST.L=%F
004 50043 1FFE 00 JUMP * IF TST.L=%T
005 30003 0D6F F8 ROT JUMP BEGIN;ROTATE; rotate top 3 stack elements
END
0 ERROR(S) DETECTED
Figure 16. LEASMB Design example: Demonstration program listing
MUXCTL
Figure 16 shows a small portion of microcode created
to load data from the Logic Engine display panel switch register
and test the stack rotate operation.
This code includes all the
necessary declarations and microinstructions to support our example,
and uses a variety of notations to illustrate features of the
LEASMB microprogram assembler.
The
SIZE
directive specifies the number of command
bits in the microinstruction (23 in our example).
Command bit fields are defined with the
COM
directive.
In Figure 16, the definition of
MUXCTL
provides the following information: MUXCTL is a field
of 11 bits, which we choose to refer to in our program with indices
running from 10 (for the leftmost bit) to 0.
In the command bit area of the microinstruction,
MUXCTL occupies command bits 4 through 14.
Thus
MUXCTL(10) occupies command bit 4 of the microinstruction.
We have chosen to describe the control in terms of logic (true
or false), rather than in terms of voltages.
In the definition of
MUXCTL,
we specify that, for each bit, truth is to be implemented
as a high voltage (T=$7FF, hexadecimal 7FF).
Further, we declare that, whenever any bit or bits of
MUXCTL
are not specifically
referenced in a particular microinstruction, the default values
for the bits are true logic levels (D=%TTTTTTTTTTT).
In many instances, we wish to deal with the set of
command signals that controls a particular multiplexer in Figure 16.
For our convenience, with the next three lines of the program
we define three variables M0, M1, and M2.
M0 is declared to be
a field of three bits, numbered 2 to 0,
which is equivalent (EQU)
to bits 9 to 7 of MUXCTL. M1 and M2
are declared similarly to
be equivalent to bits 6 to 4 and bits 3 to 2 of MUXCTL.
With these definitions, we may refer to the field
MUXCTL as a whole, or to subfields M0, M1,
and M2, or to any bit in any of the fields.
In similar fashion, we declare the attributes of
the collection of stack control signals REGCTL,
and a supporting symbol LOAD3.
In examining Figure 16, you will see that, in order
to select stack element S0 as the output of multiplexer 1, we
must supply the code 3 (binary %011)
into the M1 select inputs.
For convenience, we define a symbol M1S0 that will invoke
(INV) the value 3 on the field M1.
If in a microinstruction we wish
to pass element S0 through multiplexer 1, we may simply write
M1S0,
thus assigning the value 3 (%011) to the field M1 in the
microinstruction.
In the microcode in Figure 16, the instruction
at location 002 illustrates this usage.
The definition of the symbol ROTATE shows how we
may easily develop complex invocations.
The use of ROTATE in
the instruction at location 005 invokes the previously-defined
invocations M0S2, M1S0, and M2S1,
and invokes the value LOAD3
in the command bits defined for REGCTL.
The advantage of such
symbolic notations is that, when producing the microcode, we do
not need to be concerned about the detailed location and values
of the signals; the assembler will fill in the proper bits for
us.
The declaration of the structures for the test input
multiplexer appears at the end of the declaration phase in Figure 16.
INMUX, a field of 4 bits, defines the position in the command
bits of the multiplexer controls.
LD.L and TST.L describe values
to be invoked upon the INMUX field, as discussed below.
The illustrative microprogram in Figure 16 performs
two debugging operations: loading the contents of the display
panel switch register into stack element
S0 (and pushing the stack),
and performing a rotation of the top three elements of the stack.
Two pushbuttons on the display panel,
LD and TST, control the actions.
When LD is pressed and released, the load and push operation
will occur; pressing and releasing TST will enable the rotate
operation.
For each button, it is necessary to assure that the
microcode performing the loads and rotates will be executed only
once per button push.
To accomplish this, the code at locations
000 and 001 performs a single pulser function for
the LD button
[Winkel and Prosser, The Art of Digital Design,
Prentice-Hall, Inc., 1980, Chapter 6].
The code at locations
003 and 004
performs a similar function for the TST button.
The '*'
in the microinstruction operand fields means "value
of the assembler's location counter."
In the declaration phase, we have specified that
a use of TST.L, such as at locations
003 and 004, should invoke
the value 1 for command field INMUX; LEASMB will take care of
generating the proper values for the test multiplexer select lines.
Using information supplied in the declarations and in a given
microinstruction, LEASMB will determine the logic level entering
the 2910 sequencer's test signal input that should cause a jump,
and will translate this logic level into the proper voltage.
For instance, the microinstruction at location 003 implies that
a jump should occur if TST.L is false.
The definition of TST.L
states that the input test signal selected by TST.L has truth
represented as a low voltage (T=%L).
Thus LEASMB will conclude
that, in order to jump at location 003,
the incoming test signal
must be a high voltage level.
With this informal description of the language, you
should be able to follow the test program.
We hope that you will
appreciate how LEASMB can help define useful structures and suppress
unwanted detail.
In the next sections, the LEASMB language is
presented in full.
5.6.1
Names
Symbolic names in LEASMB may contain upper case or
lower case letters, numerals, `_', or `.'
The first character must be a letter.
Names may be any length.
Upper case letters are distinct from lower case letters.
The following symbols are reserved, and must not be used as symbolic
names:
5.6.2
Subscripts
A subscript is one of the following forms:
<subscript> := (<index1>) | (<index1>:<index2>)
where <index1> and <index2> are expressions
with numeric values.
Subscripts are used with command variables
and as command bit range specifiers.
5.6.3
Types of names
In LEASMB, a symbolic name may represent a constant
name, program label, command variable, or invocation variable.
A constant name, defined with the EQU statement,
may be of type numeric constant, voltage constant, or logic constant,
governed by the type of the defining expression.
Depending on
the context, a numeric constant may be treated as a number, a
voltage value, a logic value, or a program label.
A program label, which names a location in
the microcode, is usually defined by its appearance in the label
field of a microinstruction statement.
It may also be defined
using the EQU and ORG statements.
A command variable describes a field of contiguous
command bits in the microinstruction.
It is usually defined with
the COM statement,
but may also be defined with the EQU statement.
It may appear as a subscripted or unsubscripted symbolic name:
<command variable> := <name> | <name><subscript>
The unsubscripted form implies a range of command
bits, as determined by the context.
The subscripted form with
a single index refers to exactly one command bit; the subscripted
form with two indices refers to a range of command bits.
Examples of command variable names are:
X.REG(3:7)
PROD
Headload.L(2)
ALUCTL(5:0)
An invocation variable describes a particular
pattern to appear in specified command bits in the microinstruction.
It is defined with the INV statement or with the EQU statement,
and is a simple unsubscripted name.
5.6.4
Constants
Constants may be numeric (expressed in decimal, hexadecimal,
or binary), voltage, or logic.
There is also one special program
label constant.
A decimal numeric constant appears as a number
between 0 and 65523.
Example: 2544.
A hexadecimal numeric constant appears as
a number between 0 and FFFF, preceded by a "$" character.
Example: $4FA.
A binary numeric constant appears as a string
of 1's and 0's, preceded by "%".
The maximum usable
length of a binary constant is 16 bits.
Example: %111010.
A voltage constant appears as a string of
up to 16 "H" (for high voltage) or "L" (for
low voltage) characters, preceded by "%".
Within LEASMB,
"H" is maintained as a 1, and "L" as a 0.
Example: %HHLHLLL.
A logic constant appears as a string of up
to 16 "T" (for true) or "F" (for false) characters,
preceded by "%".
Within LEASMB, "T" is maintained
as a 1, and "F" as a 0.
Example: %FTFFFFTTT.
An asterisk "*" in the operand field of
a microinstruction means current value of the LEASMB
microinstruction location counter,
and is of type program label.
5.6.5
Expressions
Expressions may involve the arithmetic operators
"+" (add) and "-" (subtract), and the
logical operator "/" (one's complement).
Any numeric,
voltage, or logic constant may be preceded by "/" to
generate the logical complement of the constant.
Expressions
are evaluated in strict left-to-right order, with no
operator precedence.
Grouping parentheses are not permitted in
LEASMB.
LEASMB maintains constants as 16-bit unsigned quantities.
LEASMB does not distinguish between negative quantities and large
positive quantities (greater than 32767).
To avoid unwanted results,
the programmer should arrange for all expressions to evaluate
to positive quantities.
LEASMB evaluates expressions involving arithmetic
"+" and "-" as shown in Table 1.
From Table 1, we note the following:
(a)
| No operations are allowed on command variables
or invocation variables.
|
(b)
| Voltage and logic types may not be mixed in an expression.
|
(c)
| A numeric type, if combined in an expression
with an operand of another type, will take that operand's type.
|
(d)
| Arithmetic is done in two's complement notation;
"-" does not perform the logical (one's) complement
function, which is performed by "/".
|
Table 1. Results of expression evaluation
Note:
"*" designates the most useful forms of expressions.
1st operand | Operator | 2nd operand | Result
|
--- | + or - | numeric | numeric*
|
--- | + or - | voltage | voltage
|
--- | + or - | logic | logic
|
numeric | + or - | numeric | numeric*
|
numeric | + or - | voltage | voltage
|
numeric | + or - | logic | logic
|
numeric | + | program label | program*
|
numeric | --- | program label | (illegal)
|
voltage | + or - | numeric | voltage
|
voltage | + or - | voltage | voltage
|
voltage | + or - | logic | (illegal)
|
voltage | + or - | program label | (illegal)
|
logic | + or - | numeric | logic
|
logic | + or - | voltage | (illegal)
|
logic | + or - | logic | logic
|
logic | + or - | program label | (illegal)
|
program label | + or - | numeric | program*
|
program label | + or - | voltage | (illegal)
|
program label | + or - | logic | (illegal)
|
program label | + | program label | (illegal)
|
program label | - | program label | numeric
|
5.6.6
LEASMB program structure
Structure of the source program file -
A program is contained in a DOS text file, usually
prepared using a text editor.
A program consists of a sequence
of statements.
The statements are partitioned into two phases:
In the declaration phase, the programmer describes the
structure of the microinstruction and declares symbolic names
to describe components of the structure.
In the program phase,
which, if present, must follow the declaration phase, the programmer
writes the microinstructions that will form the object code destined
for the Logic Engine writable control store.
Structure of source program statements -
Each statement must begin on a new line.
Statements
consist of three major fields: label, operation, and operand.
Major fields are separated by one or more blanks.
If a statement
has a label field, it must begin in column 1; otherwise, column
1 must be blank.
Within numeric or symbolic elements, no blanks
should appear.
Elements must be separated by suitable punctuation,
which may be one of the characters , ; = or blank.
Elements may
be preceded or followed by any number of blanks.
With these provisos,
the statement format is free-field.
Although not required
by LEASMB, you will usually wish to line up the operation fields,
to improve the appearance of your listing.
Comments -
Comment lines, beginning with an asterisk "*"
in column 1, may appear preceding or following any statement.
They appear on the output listing, but are otherwise ignored
by LEASMB.
Comments may also be included at the end of any statement.
Such comments must be separated from the statement's major fields
by a semicolon.
5.6.7
LEASMB assembly directives
In the declaration phase:
|
ID | Specifies the program name
|
SIZE | Specifies the number of command bits
|
MODE | Specifies logic or voltage mode
|
COM | Defines a command bit field
|
INV | Defines an invocation variable
|
EQU | Equates a symbolic element to a value
|
In the program phase:
|
PROG | Specifies the start of the program phase
|
ORG | Specifies the origin for next section of
object code
|
EQU | Equates a symbolic element to a value
|
In either the declaration or program phase:
|
END | Specifies the end of the symbolic source
program
|
TITLE | Specifies a title to appear at the head
of each page
|
5.6.8
Description of LEASMB statements
Each LEASMB assembly directive defines a statement
of the same name, with the assembly directive's mnemonic appearing
in the operation code field of the statement.
ID statement:
The operand field is a symbolic name representing
the name of the program.
One ID statement may appear anywhere in the declaration phase.
The program name in the ID statement
appears in the object file.
If the program has no ID statement,
then the object file will contain a name of blanks.
SIZE statement:
The operand field is an expression that evaluates
to an integer between 0 and 39.
This integer is the number of
command bits in the microinstructions to be created by LEASMB.
The SIZE statement, if present, must appear prior to
any COM, INV, or EQU statement.
If no SIZE statement appears,
LEASMB assumes that the number of command bits is 0.
MODE statement:
The operand field consists of the word LOGIC
or the word VOLTAGE
In several assembly contexts,
numeric values may represent either logic or voltage values.
This statement declares the mode for numeric values whose mode
is not otherwise specified by the context.
One MODE statement
may appear anywhere in the declaration phase, and applies to the
entire program.
In the absence of a MODE statement, LEASMB uses
a mode of LOGIC.
COM statement:
The COM statement allows the programmer to define
the nature of a contiguous field of command bits.
The form is:
[<command variable>] COM <command
bit range> [,<voltage values>] [,<default values>]
A label field is optional, and if present represents
a simple or subscripted <command variable>.
The operand
field begins with <command bit range>, a subscript specifying
a command bit or a range of command bits.
The values of the <command bit range>
indices must be from 0 to 39, and if the subscript
indicates a range, then the second index cannot be smaller than
the first index.
The range size of the <command bit field>
must be no greater than 16.
For this discussion, call the size
of the range N (N is 1 to 16).
If the <command variable>
in the label field is unsubscripted, then it is assumed to have
a range of size N, with index values of 0 through N-1.
If
the <command variable> is subscripted, then the declared
size of the subscript range must be N, agreeing with the range
size of <command bit range>.
The initial (leftmost) index
of <command variable> corresponds to the initial (leftmost)
index of <command bit range>.
Subsequent index values of
<command variable> may increase or decrease, depending on
whether the second index is greater or less than the first index.
All indices must be positive.
Any symbols encountered in the
operand field must have been previously defined.
Consider two illustrations:
VAR1 COM (4:9)
VAR2(32:29) COM (12:15)
VAR1 refers to command bits 4 through 9 (a range
size of 6 bits). VAR1 has implied indices of 0 through 5, with
VAR1(0) corresponding to command bit 4.
VAR2 refers to command
bits 12 through 15 (4 bits), and has an explicitly stated range
of 32 through 29 (decreasing), with VAR2(32) corresponding to
command bit 12, VAR2(31) to command bit 13, etc.
The range sizes must match.
Following the command bit range may appear up to
two optional fields, in either order, each preceded by a comma.
The fields specify (a) the default values for each command bit
and (b) the voltage values for assertion (truth) for each command
bit.
<voltage values>
for asserting each bit are specified by T= followed
by an expression that evaluates to a numeric or voltage value.
A numeric value is taken to be a voltage specification, regardless
of the MODE declaration.
An alternative notation, for specifying
the negation (false) values for each bit, uses F=
instead of T=.
If the <voltage values> field
is absent, LEASMB assumes that assertion of each bit requires
a high voltage.
<default values>
are specified using the notation D= followed by an
expression that evaluates to a numeric, voltage, or logic constant.
If the expression is numeric, then the value takes on the type
specified by the MODE statement.
If <default values> is
absent, then LEASMB assumes that the default for each bit is a
low voltage for VOLTAGE mode,
and is the voltage for a false logic
level for LOGIC mode.
In a microinstruction, LEASMB will use
the default values for any command bits that are not specifically
referenced.
The programmer should take care not to overlap
<command bit range>s in COM declarations,
since such overlap can cause
ambiguities as to the correct default and voltage values.
(The
programmer can create alternative names for command bit fields
and subfields with the EQU statement.)
LEASMB views the value of the <default values>
and <voltage values> expressions as binary numbers, and
assigns the least significant bit to the rightmost bit of <command
bit range>.
Extra significant bits on the left of those required
to fill the field are discarded, but will cause a warning message.
Here are some examples of command statements:
VAR3(6:0) COM (0:6),D=%1011100,T=%1110000
INPUTMUX(3:0) COM (11:14),D=%TTFF
REGLOAD COM (15:16),D=2,T=%LL
HALTFF.SET COM (10)
The effect of these definitions is summarized in
the following table:
Variable
| Variable indices
| Command bits
| Mode VOLTAGE Truth
| Mode VOLTAGE Default
| Mode LOGIC Truth
| Mode LOGIC Default
|
VAR3
| 6 to 0
| 0 to 6
| HHHLLLL
| HLHHHLL
| HHHLLLL
| HLHLLHH
|
INPUTMUX
| 3 to 0
| 11 to 14
| HHHH
| HHLL
| HHHH
| HHLL
|
REGLOAD
| 0 to 1
| 15 to 16
| LL
| HL
| LL
| LH
|
HALTFF.SET
| 0
| 10
| H
| L
| H
| L
|
We recommend that you study the table carefully, so that you fully
appreciate the power and use of the notations at your disposal.
In using command variables in other statements, you may use a
subscripted or unsubscripted form.
An unsubscripted usage implies
the entire defined range of the variable.
In a subscripted usage,
the specified range must be completely within the range defined
for the command variable.
INV Statement:
The INV statement is used to define an invocation variable which,
when used in a microinstruction, will cause a specified value
to be imposed on specified command bits.
This is an exceedingly
powerful notation, and is the key to programming in a structured,
disciplined manner.
The INV statement has this form:
<invocation variable> INV <specification list> [,<input
truth value>]
Any symbols appearing in the operand field must be previously
defined.
The <invocation variable> is a simple, unsubscripted
name.
The <specification list> consists of one or more
<specification>s, separated by commas.
Each <specification>
is one of the following forms:
<specification>
| := | <command bit range>
|
| | <command variable>
|
| | <command bit range>=<code>
|
| | <command variable>=<code>
|
| | <invocation variable>
|
The form <command bit range> implies that, when the invocation
variable is used, the specified command bits will be asserted.
<command variable> may be subscripted or unsubscripted,
and the form implies that the specified command bits will be
asserted.
An unsubscripted <command variable> implies the entire
defined range for that variable.
<command bit range>=<code> implies that the specified
command bits will receive the voltages specified by <code>.
<code> is an expression that evaluates to a numeric value
(with logic or voltage mode implied by the MODE declaration),
a logic value, or a voltage value.
<command variable>=<code> implies that the specified
command bits will receive the voltages specified by <code>.
<code> is an expression that evaluates to a numeric value
(with logic or voltage mode implied by the MODE declaration),
a logic value, or a voltage value.
<invocation variable> implies that the invocation specified
in the definition of that variable will be performed.
Consider the following declarations:
VAR4 COM (5:9),T=%HLHHL
VAR5 COM (10:13),T=%LLHH
COM (0:3),T=%HLHL
INV1 INV (6:8)
INV2 INV VAR4=%HHHLL
INV3 INV VAR4(0:4)
INV4 INV (0:3)=%1100
INV5 INV VAR5(1:3)=%TFT
INV6 INV INV1,VAR5=%0110,INV4
The effect of the invocation statements is presented in the following
table:
Invocation Variable
| Command bits affected
| Mode VOLTAGE Command bits
| Mode LOGIC Command bits
|
INV1 | 6 to 8 | LHH | LHH
|
INV2 | 5 to 9 | HHHLL | HHHLL
|
INV3 | 5 to 9 | HLHHL | HLHHL
|
INV4 | 0 to 3 | HHLL | HLLH
|
INV5 | 11 to 13 | LLH | LLH
|
INV6 | 6 to 8 10 to 13 0 to 3 | LHH LHHL HHLL | LHH HLHL HLLH
|
The programmer should take care not to refer to overlapping command
bit ranges in an INV statement, as LEASMB may produce unpredicted
results.
<input truth value> in invocation statement:
The 2910 can accept one input signal for testing to help determine
which 2910 instruction option -- pass or fail --
is executed.
The Logic Engine requires that this signal appear
on the Designer's Condition Code input.
Although invocations
have quite general application in microcode development, frequently
the designer will wish to use an invocation to select the particular
signal to appear on Designer's Condition Code.
The invoked code
selects that particular signal from among the designer's collection
of possible test input signals.
The mechanism by which the selection
occurs is the designer's responsibility; the selection apparatus
may use a multiplexer, three-state bus, or other method,
controlled from the Logic Engine microcode with an invocation
variable.
Each potential input test signal has its own code.
With <input truth value>, LEASMB allows the programmer to
specify the logic convention for the particular invoked signal.
<input truth value> has the form:
<input truth value> :=
T=<voltage expression>
|
F=<voltage expression>
where <voltage expression> evaluates to a numeric value
(with implied VOLTAGE mode) or a voltage value.
If
<input truth value> is absent, LEASMB assumes a T=%H convention.
Note that the <input truth value> has no direct connection
with the <code>; <input truth value> describes the
logic convention for a signal that will presumably appear on Designer's
Condition Code when the particular code is invoked in the specified
command bits.
Examples of <input truth value> usage:
TESTSIG.a INV (15:18)=%LLHL,T=%L
TESTSIG.b INV (15:18)=%LLHH,T=%H
---
JUMP ABC IF TESTSIG.a=%F
JUMP XYZ IF TESTSIG.b=%T
The first JUMP microinstruction requires a jump to location ABC
if TESTSIG.ais false at the time of execution of the microinstruction.
The definition of TESTSIG.adeclares that the incoming test signal
has T=%L, so false is a high voltage.
LEASMB will create proper
object code to invoke TESTSIG.a(with voltages %LLHL on
command bits 15 to 18), and will recognize that a high voltage
on Designer's Condition Code is required for the jump to occur.
In similar manner, the second JUMP microinstruction requires truth
on TESTSIG.b for the jump to occur.
LEASMB will invoke TESTSIG.b
(with voltages %LLHH on command bits 15 to 18), and will arrange
for a high voltage on Designer's Condition Code to cause a jump.
These powerful LEASMB structures can free the programmer from
many tedious details of bit positions, codes, and voltage values,
allowing the designer to concentrate fully on the higher-level
aspects of the control program.
EQU Statement -
The EQU statement allows the programmer to define values for names,
and to equate variable names.
For assigning values to names,
the form of the statement is:
<name> EQU <expression>
Any symbols appearing in the expression must be previously defined.
The <name> must be a simple, unsubscripted name.
The <expression>
must evaluate to a numeric, logic, voltage, or program label value,
which will become the value and type of the specified <name>.
Thus this form of EQU
can define <constant name>s and <program label>s.
For equating variable names, the form of the EQU statement is:
<variable> EQU <specifier>
Any symbols appearing in the operand field must be previously
defined. <variable> may be a simple or subscripted name.
The forms allowed for <specifier> and the type of the resulting
<variable> are:
Form
| Produces
|
<specifier> := <command bit range>
| <command variable>
|
<command variable>
| <command variable>
|
<invocation variable>
| <invocation variable>
|
For a command variable definition, a subscript range on the defined
variable may be explicitly provided, in which case the range size
must match the range size of the specifier.
If a range is not
specified, then the defined command variable assumes the same
range size as the specifier, with indices starting at 0.
Invocation
variables may not be subscripted.
Within the definition phase of a source file, EQU may be
used to define numeric, logic, or voltage constants, and to create
command and invocation variables.
Within the program phase of a source file, EQU may be used
to define numeric constants or program labels.
The following statements contain illustrations of valid EQU statements:
VAR10 COM(10:20)
INV10 INV VAR10=$1D
TURNON EQU %HLLHL
ACCLR EQU $10
SUB1(3:0) EQU VAR10(0:3); SUB1 is a 4-bit subfield of VAR10
SAME10 EQU INV10
---
CONT
ABC EQU *+2; ABC is location of CRTN instruction + 2
CRTN
PROG statement:
The PROG statement must be the first statement in the program
phase of the source file.
It marks the end of the declaration
phase and the beginning of the program phase.
It has no label
field or operand field.
ORG statement:
The ORG statement sets the LEASMB microinstruction location counter,
and thus defines the writable control store address of the next
microinstruction in the program. The form of the ORG statement
is:
[<program label>] ORG <expression>
The required operand field is an expression of type numeric constant
or program label.
LEASMB truncates the value of the <expression>
to 12 bits, and issues a warning message if significant bits are
lost.
A label field is optional; if present, the symbolic name
will be given the value of the operand field expression, with
type program label.
If ORG sets the location counter backwards, the programmer may
(intentionally or unintentionally) overlay previous microinstructions.
The last usage of an address will prevail.
LEASMB does not issue
an error or warning message.
END statement:
The END statement is the last statement in the program.
It marks
the end of the program phase, if any, or the end of the declaration
phase if there is no program phase.
It has no label field or
operand field.
TITLE statement:
The TITLE statement allows the programmer to specify a title that
will appear at the head of each new page after the occurrence
of the TITLE statement.
The title begins with the first non-blank
character in the operand field, and may be up to 74 characters
in length.
The TITLE statement must be contained on one source
line.
A blank or void title will disable the titling feature.
TITLE statements may appear anywhere in the source program; they
do not themselves appear in the listing, and do not cause a page
eject.
TITLE statements have effect only if the assembly page
option is operating.
The MICROINSTRUCTION statement:
Each microinstruction statement in the source program results
in the production of one object code microinstruction. LEASMB
assigns microinstructions to consecutive locations in writable
control store, starting with 0, unless directed otherwise with
the ORG assembly directive.
The microinstruction statement has
the form:
[<program label>] <sequencer field> [;<command list>]
If a comment appears at the end of a microinstruction statement,
then a command list must be present, even if it is null.
Thus,
if a comment is present, two semicolons appear, one marking the
start of the (possibly null) command list, and the other marking
the start of the comment.
The <sequencer field>
allows the programmer to direct the activities
of the 2910, the device whose task is to produce the address of
the next microinstruction.
The 2910 instruction set consists
of sixteen basic operations, each with two options, pass
and fail, governed by the status of 2910 inputs CCEN.L
and CC.L.
The LEASMB <sequencer field> provides the ability
to exert complete control of the 2910.
As you study this section,
you may wish to refer to the sample program that appears in section
XXX.
The structure of <sequencer field> is:
<sequencer field> := <operation> [<D-field>]
[IF <test condition>]
<operation> is one of the standard 2910 I-field
mnemonics, or one of a set of alternative mnemonics defined within
LEASMB.
Allowable forms are:
<operation> :=
| <I-field mnemonic>
|
| <I-field mnemonic>,PASS
|
| <I-field mnemonic>,FAIL
|
where the latter two forms will force the 2910 to execute its
pass or fail option, respectively.
Table 2 shows the 2910 I-field
mnemonics recognized by LEASMB.
Table 2. 2910 Instructions.
I-field value
| Mnemonic
| Function
|
Standard 2910 mnemonics
|
$0
| JZ
| Jump to location 0
|
$1
| CJS
| Conditional jump to subroutine at PL address
|
$2
| JMAP
| Jump to map address
|
$3
| CJP
| Conditional jump to PL address
|
$4
| PUSH
| Push with conditional load of counter
|
$5
| JSRP
| Jump to subroutine at R address or at PL address
|
$6
| CJV
| Conditional jump to vector address
|
$7
| JRP
| Conditional jump to R address or PL address
|
$8
| RFCT
| Repeat loop if counter is non-zero
|
$9
| RPCT
| Jump to PL address if counter is non-zero
|
$A
| CRTN
| Conditional return from subroutine
|
$B
| CJPP
| Conditional jump to PL address and pop
|
$C
| LDCT
| Load counter and continue
|
$D
| LOOP
| Test end of loop
|
$E
| CONT
| Continue
|
$F
| TWB
| Three-way branch
|
Alternate forms supported be LEASMB
|
$3
| JUMP
| Equivalent to CJP
|
The programmer may specify the contents of the 2910 D-field.
The optional <D-field> is an expression of
type numeric constant or program label.
The expression is truncated
to 12 bits; if significant bits are dropped, LEASMB issues a warning
message.
If <D-field> is absent, LEASMB inserts the value 0.
The optional IF <test condition> field
allows the programmer to control the choice of pass or fail option
within the 2910.
Refer to the earlier discussion of the INV Statement.
<test condition> has one of these forms:
<test condition> := <invocation variable>
|
<invocation variable>=<test input value>
where <test input value> is an expression that evaluates
to a one-bit numeric (1 or 0),
voltage (H or L), or logic
(T or F) constant.
(Expression values larger than one bit are
truncated, with a warning message.)
The purpose of <invocation variable> is to issue the proper
command bit values to select a desired test input signal from
the designer's hardware.
<test input value> allows the programmer to specify the
value of the test input signal that will cause the 2910 to execute
its pass option.
If the =<test input value>
phrase is absent, then LEASMB assumes the value T.
As an illustration, consider the following definitions and microinstruction
statements:
ACZERO INV (4:7)=%1011,T=%H
COMPARE INV (4:7)=%0010,T=%L
...
CJP XYZ IF ACZERO = %F
CRTN IF COMPARE
The use of ACZERO in the CJP instruction invokes the value %1011
(= %TFTT = %HLHH) onto command bits 4 through 7.
The designer
should arrange that this pattern in command bits 4 through 7 will
cause a signal ACZERO to appear at the Designer's Condition Code
input.
Further, the declaration states that when this occurs,
the incoming test signal uses a high voltage for truth.
In the
CJP instruction, the jump is to occur if
ACZERO is false (a low voltage).
LEASMB will arrange that a low voltage delivered at
the Designer's Condition Code input will cause the jump to occur.
In the second example, the use of COMPARE causes the value
%0010
(= %FFTF = %LLHL)
to appear on command bits 4 through 7, which
we infer is going to cause a signal COMPARE
to appear at the Designer's
Condition Code input.
The 2910 will execute a Return (the pass
option for CRTN) if this incoming signal is true.
Since the definition
of COMPARE states that truth is a low voltage, LEASMB will arrange
that the pass option will occur when a low voltage appears at
the Designer's Condition Code input.
The IF <test condition> phrase will have no
effect if used in a microinstruction statement in which the programmer
has used the explicit PASS or FAIL form of <operation>.
The simple form <I-field mnemonic> without the
IF <test condition>
phrase will cause the 2910 to execute its pass option.
The following table summarizes the programmer's
control of the 2910's pass and fail options:
Form
| Result
|
<I-field mnemonic>
| Pass
|
<I-field mnemonic>,PASS
| Pass
|
<I-field mnemonic>,FAIL
| Fail
|
<I-field mnemonic>...IF <test condition>
| Governed by Designer's Condition Code input
|
The Microinstruction Command List:
In the command list, the programmer specifies signal values for
command bits.
The purpose of the entire microprogram is to deliver
an orderly sequence of command bit values to the designer's architecture.
<command list> consists of a list of <command specification>s,
each separated by a comma, where each <command specification>
has one of these forms:
<command specification> :=
| <command bit range>
|
| <command variable>
|
| <command bit range>=<field value>
|
| <command variable>=<field value>
|
| <invocation variable>
|
Note that these structures are equivalent to those permitted in
defining an invocation variable.
The form <command bit range> implies that the specified
command bits will be asserted.
<command variable> may be subscripted or unsubscripted,
and the form implies that the specified command bits will be
asserted.
<command bit range>=<field value> implies that the
specified command bits will receive the voltages specified by
<field value>.
<field value> is an expression that
evaluates to a numeric value (with logic or voltage mode implied
by the MODE declaration), a logic value, or a voltage value.
<command variable>=<field value> implies that the
specified command bits will receive the voltages specified by
<field value>.
<field value> is an expression that
evaluates to a numeric value (with logic or voltage mode implied
by the MODE declaration), a logic value, or a voltage value.
<invocation variable> implies that the invocation specified
in the definition of that variable will be performed.
In the object code for a microinstruction, all command bits not
specifically addressed in a command specification will receive
their default voltage values.
STRUCTURE OF A LOGIC ENGINE OBJECT CODE MICROINSTRUCTION
An LEASMB microinstruction consists of a fixed-format sequencer
field (20 bits) and a command bit field of size specified by the
designer.
The structure of the sequencer field is as follows (bits
are numbered from left to right, beginning with bit 0):
Field
| Bits
| Name
| Description
|
I (4 bits):
| 0-3
| I-field
| 2910 instruction code field (high-active)
|
X (4 bits):
| 4
| CCEN.L
| 2910 Condition Code Enable (low-active)
|
| 5
| CIN:
| 2910 Carry In (high-active)
|
| 6
| CCFAIL:
| Condition Code Fail (high-active)
|
| 7
| CCINV:
| Condition Code Invert (high-active)
|
D (12 bits):
| 8-19
| D-field
| 2910 address field (high-active)
|
B:
| 20
|
| Breakpoint (high active)
|
The structure of the command bit field is:
Bit | 24 | 23 | 22 | .... | 62 | 63
|
Command Bit | 0 | 1 | 2 | .... | 38 | 39 (maximum)
|
The D-field is specified by the programmer as an expression
of type program label or numeric constant.
The I-field is generated by LEASMB from the mnemonic in the
operation code field of the microinstruction.
The B-field is generated by LEASMB when a breakpoint is requested
for that microinstruction.
If breakpoints are enabled, the clock
will be stopped before the execution of a microinstruction that
has this bit set.
The X-field:
The bits in the X-field allow the programmer to exert, through
the prescribed LEASMB syntax, detailed control over certain of
the 2910 microprogram sequencer features.
In normal programming,
LEASMB will set the X-field bits automatically, and the programmer
will not deal with them.
The use of the X-field is explained below.
The discussion assumes you are familiar with the operations
of the 2910.
For each of its instructions, the 2910 sequencer provides two
sub-operations, PASS and FAIL.
The 2910 performs the PASS
sub-operation if its CCEN.L input is high (false) or if its
CC.L input is low (true).
The 2910 performs the FAIL sub-operation
under other conditions, namely, if its CCEN.L input is low (true)
and its CC.L input is high (false).
A designer may wish to control
the choice of sub-operation by forcing a PASS, forcing a
FAIL, or allowing his Designer's Condition Code input to determine
the choice.
The Logic Engine microinstruction provides the means
to select each of these alternatives easily.
The designer may force the 2910 to select the PASS sub-operation
by setting the CCEN.L bit to 1 (false) in the microinstruction.
(LEASMB performs this bit setting automatically if you specify
the PASS option in the sequencer field of a symbolic microinstruction,
or if you fail to specify a conditional clause.)
The designer may force the 2910 to select the FAIL sub-operation
by setting the CCEN.L bit to 0 (true) and the CCFAIL bit to 1
(true) in the microinstruction.
(LEASMB performs this bit setting
automatically if you specify the FAIL option in the sequencer
field of a symbolic microinstruction.)
The designer may allow his Designer's Condition Code signal to
control the choice of PASS or FAIL sub-operation by setting
the CCEN.L bit to 0 (true) and the CCFAIL bit to 0 (false) in
the microinstruction.
(LEASMB performs this bit setting automatically
if you specify the conditional IF clause in a symbolic
microinstruction.)
Here is a summary of the bit values required to influence the
choice of sub-operation:
| CCEN.L
| CCFAIL
|
Force a PASS
| 1 (high, false)
| ---
|
Force a FAIL
| 0 (low, true)
| 1 (high, true)
|
Act on incoming Designer's Condition Code signal
| 0 (low, true)
| 0 (low, false)
|
The signal appearing on the Designer's Condition Code input may
be low-active (asserted by a low voltage) or high-active
(asserted by a high voltage).
The Logic Engine microinstruction
contains the CCINV bit to allow the programmer to specify which
voltage polarity is in effect.
To specify that Designer's Condition
Code is low-active, the designer may set CCINV to 0 (false).
To specify that Designer's Condition Code is high-active,
the designer may set CCINV to 1 (true).
(LEASMB sets CCINV automatically,
based on the declarations for the signal referenced in the IF
clause of a symbolic microinstruction.)
In the Logic Engine, the logic equation governing the signal entering
the 2910's Condition Code (CC.L) pin is:
2910.Condition.Code = (Designer's.Condition.Code EOR CCINV) *
/CCFAIL
(EOR, *, and / are the logical operators "exclusive or",
"and" and "not", respectively.)
The CIN bit of the microinstruction connects directly to the 2910
CIN pin.
Normally, this bit will be a 1 (high, true), implying
normal sequencing if a branch does not occur.
Normal sequencing
is inhibited if CIN is 0 (low, false).
The programmer may override
the normal value of CIN by placing a "/"
preceding the 2910 operation mnemonic.
Table A1. Disposition of 2910 Signals
2910 Signal
| Disposition
|
D (12 bits)
| Input. When PL.L is asserted, the Logic Engine pipeline
register provides these bits. When PL.L is negated (i.e., when
MAP.L or VECT.L is asserted), the designer's circuit must supply
these bits.
|
I (4 bits)
| Input received from Logic Engine pipeline register.
|
CC.L
| Input received from Logic Engine.
Derived from Designer's Condition Code, CCINV, and CCFAIL.
|
CCEN.L
| Input received from Logic Engine pipeline register.
|
CI
| Input received from Logic Engine pipeline register.
Programmer may specify the value through LEASMB.
|
RLD.L
| Input available at 2910 chip on Logic Engine board.
Not used by Logic Engine.
|
OE.L
| Input controlled exclusively by Logic Engine.
|
CP
| Input supplied exclusively by Logic Engine.
Designer must supply the System Clock from which CP is derived.
|
Y (12 bits)
| Output available on 60-pin flat-cable connector.
Used by Logic Engine.
|
FULL.L
| Output available at 2910 chip on Logic Engine board.
Used by Logic Engine.
|
PL.L
| Output available at 2910 chip on Logic Engine board.
Used by Logic Engine.
|
MAP.L
Output available on the 2910 PLD.
Not used by Logic Engine.
|
VECT.L
| Output available on the 2910 PLD.
Not used by Logic Engine.
|
Note: Directions are relative to the 2910.
| |
[Contents]
[Prev: 4 Logic Engine Panel]
[Next: 6 ED PLD]